Subscribe To Our NewsLetter
Share This Post:
Fibers is a new feature in PHP 8.1 that brings lightweight and controlled concurrency to PHP.
PHP 8.1 Fibers introduce efficient, non-blocking execution, making Drupal faster and more responsive. Instead of waiting for sequential HTTP requests, Fibers run them simultaneously, reducing load times and enhancing user experience. This optimization is particularly useful when working with multiple cURL-based API calls or external data fetching.
Drupal Use
Real-Time Use Case of PHP Fibers in Drupal
Since Drupal is single-threaded, we can't run true asynchronous tasks like in Node.js. However, PHP Fibers can help optimize performance by allowing tasks to be executed without blocking the main script.
Scenario: Optimize External API Calls in a Drupal Block
Let's say we have a custom Drupal block that fetches data from multiple APIs (e.g., weather, stock prices, and news) and displays it.
🔴 Problem Without Fibers (Blocking Execution)
If we make sequential API requests, we wait for each request to finish before moving to the next.
This makes the page load slow, especially if API responses take time.
🟢 Solution With Fibers (Faster Execution)
We fire all API calls at once in Fibers.
The script continues execution instead of waiting.
Once responses are ready, we resume Fibers and fetch data.
Example :
<?php
namespace Drupal\my_module\Service;
use Fiber;
use GuzzleHttp\ClientInterface;
use GuzzleHttp\Exception\RequestException;
/**
* Service for fetching API data asynchronously using PHP Fibers.
*/
class AsyncApiService {
protected ClientInterface $httpClient;
public function __construct(ClientInterface $http_client) {
$this->httpClient = $http_client;
}
/**
* Fetch multiple API responses asynchronously.
*/
public function fetchData(array $urls): array {
$fibers = [];
$results = [];
// Create a Fiber for each API request
foreach ($urls as $key => $url) {
$fibers[$key] = new Fiber(function () use ($url, $key, &$results) {
try {
$client = new \GuzzleHttp\Client();
$response = $client->get($url);
$results[$key] = json_decode($response->getBody()->getContents(), true);
} catch (RequestException $e) {
$results[$key] = ['error' => $e->getMessage()];
}
Fiber::suspend(); // Pause execution (non-blocking)
});
}
// Start all API requests in parallel
foreach ($fibers as $fiber) {
$fiber->start();
}
// Main script continues execution here without waiting for responses
\Drupal::logger('my_module')->notice('Main script running while waiting for API responses.');
// Resume all Fibers to collect responses
foreach ($fibers as $fiber) {
if ($fiber->isSuspended()) {
$fiber->resume();
}
}
return $results;
}
}
Why Use Fibers in Drupal?
Problem Without Fibers
1. API requests run sequentially, slowing down page load.
2.The page waits for each request to finish before rendering.
3. A slow API can delay the entire request.
Solution With Fibers
1. All API requests run simultaneously, reducing wait time.
2. The page loads faster, as requests run in the background.
3. Other API data is displayed while waiting for slow responses.
Conclusion
At LN Webworks, we are committed to leveraging the latest technologies to build high-performance Drupal solutions. With PHP 8.1 Fibers, we can optimize API calls, enhance speed, and create seamless user experiences by enabling non-blocking execution. By integrating Fibers into Drupal applications, we ensure faster data retrieval, better resource management, and a more efficient system overall.
Looking to enhance your Drupal website’s performance? Partner with LN Webworks and let’s build a faster, smarter, and more scalable web solution together!Â
Â
Share This Post:
Author Information
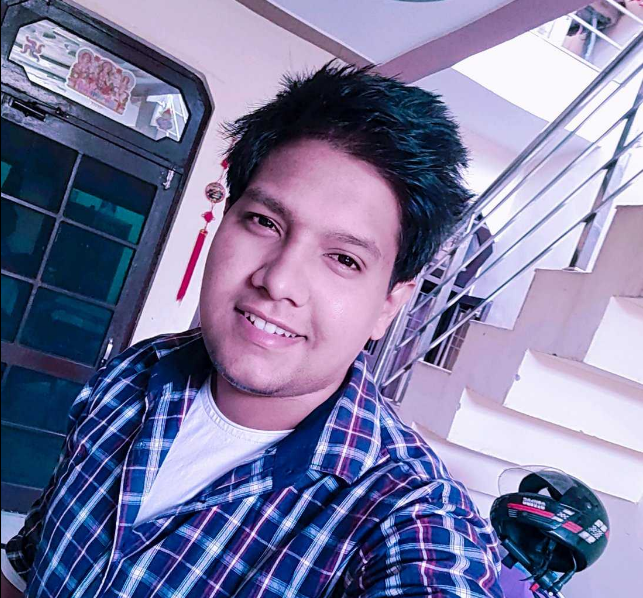
Suraj Singh
Web DeveloperChallenging tasks keep me dynamic and eager to learn, while experience builds my confidence in helping others with ease. Experienced in Drupal development, PHP, JavaScript, CodeIgniter, REST API, Git, and Drush. I believe that your achievements speak louder than words.
Talk With Certified Drupal Experts Of LN Webworks!
Related Articles
February 25, 2025
Exploring PHP 8.4: New Features and Deprecations
February 24, 2025
Best WordPress Security Plugins for 2025
February 21, 2025