Subscribe To Our NewsLetter
Share This Post:
In today’s digital landscape, ensuring the security of data transmission between clients and servers is paramount. One effective method to enhance security is by encrypting data before sending it in a request and then decrypting it on the server side. This approach provides an additional layer of security, safeguarding sensitive information from potential interception and tampering.
In this blog, we’ll explore the benefits of encrypting request data and demonstrate how to implement this in a Nextjs application using the ‘crypto-js’ library.
Why Encrypt Request Data?
Encrypting request data is crucial for protecting sensitive information during transmission over networks. It ensures data privacy by preventing unauthorized access and eavesdropping. Encryption also maintains data integrity, making it difficult for attackers to alter the information. This practice builds trust, complies with regulatory requirements, and safeguards against various cyber threats.
Protecting Sensitive Information
When data is transmitted over the internet, it can be intercepted by malicious actors. Encrypting data ensures that even if intercepted, the information remains unreadable without the correct decryption key.
Ensuring Data Integrity
Encryption helps in verifying that the data has not been tampered with during transit. Any alterations to the encrypted data will result in a failed decryption process, alerting the server to potential tampering.
Compliance with Regulations
Many industries are subject to regulations that require the protection of sensitive information, such as personal data and financial information. Encrypting data in transit can help meet these regulatory requirements.
How To Implementing Encryption in a Next.js Application
Let’s walk through an example of how to encrypt data in a request using Next.js with the App router and the ‘crypto-js’ library.
Setting Up the Next.js Application
First, if you haven’t already, set up a new Next.js project:
npx create-next-app encrypted-requests
cd encrypted-requests
Next, install the ‘crypto-js’ library:
npm install crypto-js
Encrypting Data on the Client Side
We’ll create a simple form that collects user input and encrypts it before sending it to the server.
// app/page.js
"use client";
import { useState } from "react";
import CryptoJS from "crypto-js";export default function Home() {
const [data, setData] = useState(""); const handleSubmit = async (e) => {
e.preventDefault();
const encryptedData = CryptoJS.AES.encrypt(
data,
"hysi73486jdft54W"
).toString();
await fetch("/api/dummy", {
method: "POST",
headers: {
"Content-Type": "application/json",
},
body: JSON.stringify({ data: encryptedData }),
});
};
return (
<div>
<form onSubmit={handleSubmit}>
<input
type="text"
value={data}
onChange={(e) => setData(e.target.value)}
/>
<button type="submit">Submit</button>
</form>
</div>
);
}
Decrypting Data on the Server Side
Now, let’s create an API route in Next.js that will decrypt the received data.
app/api/dummy/route.js
import CryptoJS from "crypto-js";
export async function POST(req) {
const data = await req.json();const bytes = CryptoJS.AES.decrypt(data.data, "hysi73486jdft54W");const decrypted data = bytes.toString(CryptoJS.enc.Utf8);
console.log("Decrypted data =>", decryptedData);
return Response.json({
message: "Data received and decrypted",
data: decryptedData,
});
}
Testing the Implementation
Run your Next.js application:
npm run dev
Open your browser and navigate to ‘http://localhost:3000’.
Enter some data in the form and submit it.
You should see the data being encrypted in the network request and decrypted on the server side.
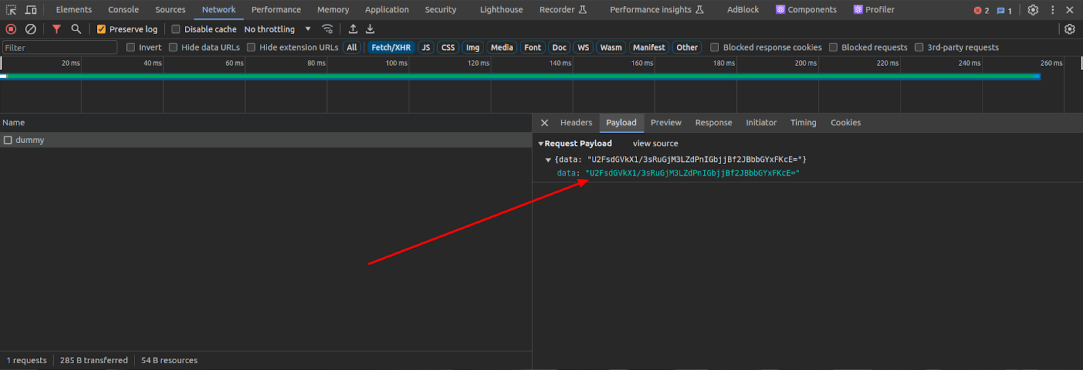
Let’s Wrap It Up!
Encrypting data in requests is a simple yet powerful way to enhance the security of your web applications. By leveraging libraries like ‘crypto-js’, you can easily implement encryption and decryption, protecting sensitive information from interception and tampering. This additional layer of security is crucial in today’s digital world, ensuring that your user’s data remains safe and secure.
At LN Webworks, our experts are always prepared to help you. Contact us today to schedule your free consultation!
Share This Post:
Author Information
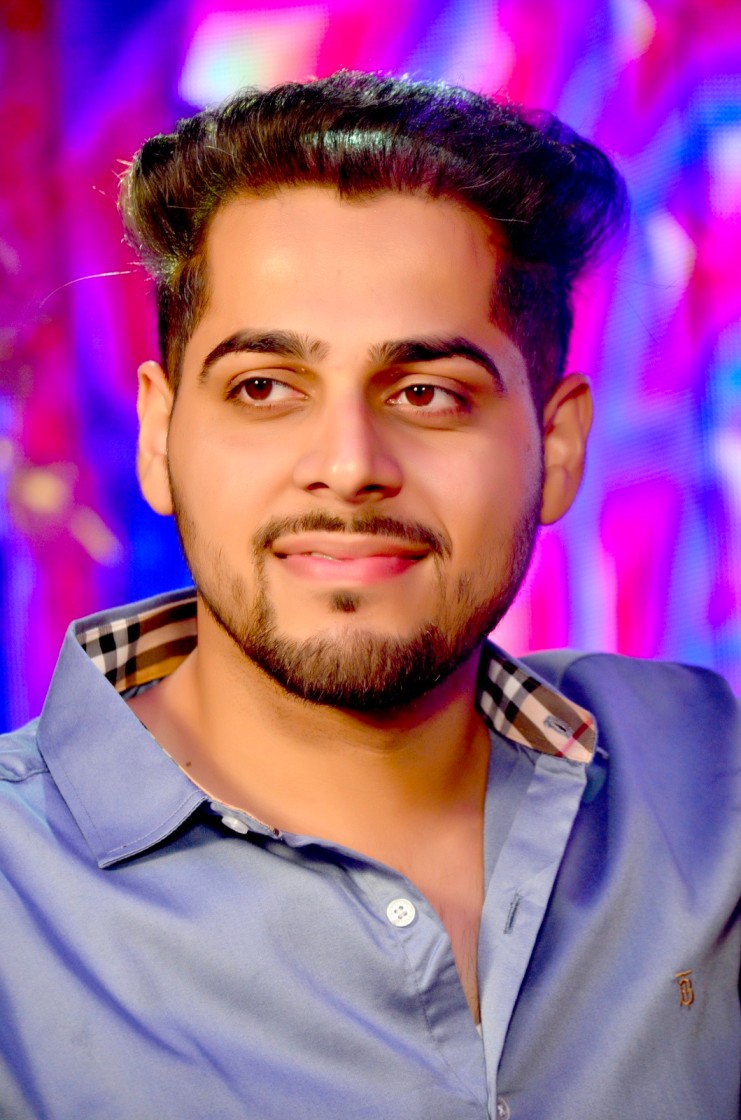
Jatin
Web DeveloperHello, my name is Jatin Duggal. I have over two years of experience as a Full Stack Web Developer, proficient in technologies such as React.js, Next.js, MongoDB, PostgreSQL, Express.js, Node.js, Git, GitHub, and AWS. I am passionate about technology and consistently seek to learn and adopt emerging technologies to enhance creativity and productivity in my work.
Talk With Certified Experts Of LN Webworks!
Related Articles
May 20, 2024
Step By Step Guide To Git Rebase Command
May 15, 2024