Have you ever felt as though your app is caught in a never-ending cycle, and you're unsure of what to do next? That's where the concept of transition states comes in. It helps your app smoothly move from one stage to the next, making sure everything keeps running without any breakage or glitches. Whether you're managing complex workflows or tracking user progress, transition states make sure your app knows exactly what to do based on what's happened before.
In this blog, we'll dive into how to implement this powerful technique in PHP using a state table. It's like giving your app a roadmap, ensuring it always knows the right turn to take. Ready to guide your app through its next adventure? Let's get started!
What Is A Finite State Machine (FSM)?
States
A Finite State Machine (FSM) is a computation model that is applied to the design of state-separate systems. Specifies States: Various points in the process (e.g., "Start," "Processing," "Completed").
Transitions
The transition from one state to another and is usually triggered by some conditions or events (e.g., "Success", "Failure").
An FSM is beneficial in workflows where the system behaves differently depending on what state it is in, and it helps in tracing processes and which kind of action should be taken next.
Why Use FSM Transition States In PHP?
In PHP, FSM transition states are helpful in managing asynchronous operations, retries, and task failures in a structured manner. Consider an application that needs to handle tasks like copying files to a remote server. The copying process may not finish instantly, so the system needs to wait and periodically check the progress.
Benefits Of FSM Transition States
Organized Workflow
FSMs provide a clear structure to your application by organizing tasks and their transitions.
Error Handling
FSMs can automatically retry tasks if they fail or move to a failure state after multiple attempts.
Asynchronous Operations
FSMs work well with asynchronous operations, such as waiting for a file copy to finish or waiting for an external API to respond.
State Tracking
You can track each task's state and know exactly where it is in the process.
Real-World Example: Handling Asynchronous Tasks
For example, you have to copy a massive dataset from one server to another asynchronously. This needs to be done in several steps, and at any step, you'll likely want to check its progress or decide to retry or fail the task. And here come FSMs.
How FSM Transition States Work In PHP?
To better understand FSMs in PHP, let's break it down with an example. In the state machine, you will define the states, transitions, and actions.
State Table Example
Here's an example state table for a file-copying operation:
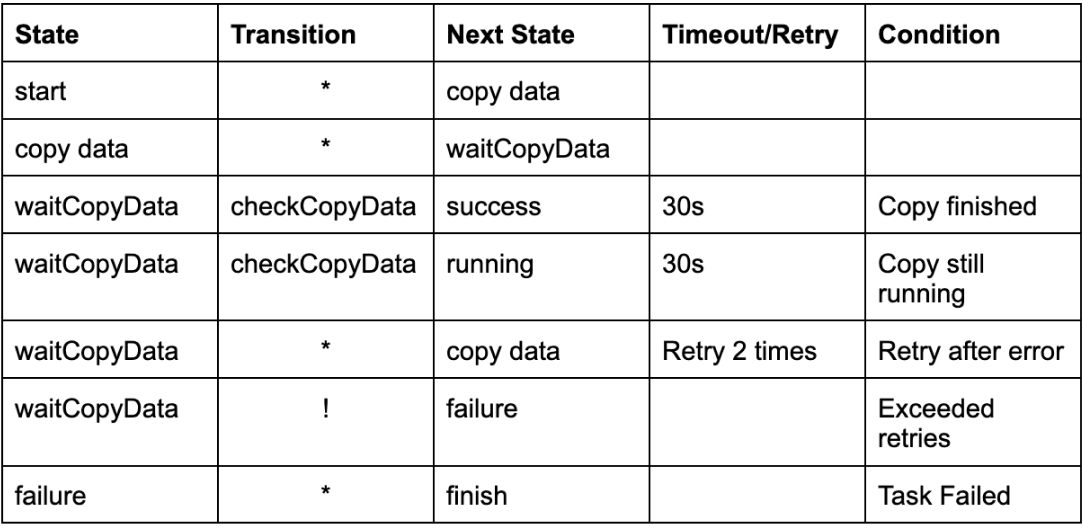
Explanation Of the States
Start State: Initializes the process and sets up necessary variables.
Copy Data State: Begins the file copy process asynchronously.
Wait for Copy Data: Checks the status of the file copy. It has transitions based on the result of the copy operation.
- If the copy is successful, the system moves to the "finish" state.
- If it's still running, the system waits for 30 seconds and checks again.
- If the operation fails, the system retries up to two more times or moves to the "failure" state after exceeding the retry limit.
Failure State: Handles errors and finalizes the operation if all retries fail.
Finish State: Completes the process, indicating success or failure
How It Works?
The state transitions depend on the outcome of the task. For example, if the file copy is still running, the system waits and retries. If it succeeds, it moves to the finish state. This ensures the system can handle errors, retries, and asynchronous tasks in a manageable way.
FSM Implementation In PHP
Let’s dive into the PHP code that implements FSM for our example of copying data.
Let’s dive into the PHP code that implements FSM for our example of copying data.
?php
class FSM {
private $state;
private $context;
private $retryCount = 0;
private $maxRetries = 2; // Maximum number of retries
private $stateTable = [];
public function __construct() {
$this->state = 'start'; // Initial state
$this->context = [];
// Define the state table with states and transitions
$this->stateTable = [
'start' => [
'*' => 'copyData' // From 'start', transition to 'copyData'
],
'copyData' => [
'*' => 'waitCopyData' // From 'copyData', transition to 'waitCopyData'
],
'waitCopyData' => [
'checkCopyData' => [
'success' => 'finish', // On success, transition to 'finish'
'running' => 'waitCopyData', // If still running, wait 30 seconds
'*' => 'copyData', // Retry if error (up to 2 times)
'!' => 'failure' // If failed after retries, go to failure
]
],
'failure' => [
'*' => 'finish' // End the process on failure
]
];
}
public function run() {
while ($this->state !== 'finish') {
echo "Current state: $this->state\n";
// Get the possible transitions for the current state
$transitions = $this->stateTable[$this->state];
// Perform the transition based on the condition
if (isset($transitions['checkCopyData'])) {
$status = $this->checkCopyData(); // Get the current status (success, running, or failure)
echo "Copy data status: $status\n";
$nextState = $transitions['checkCopyData'][$status] ?? $transitions['checkCopyData']['*'];
if ($status === 'running') {
echo "Waiting for 30 seconds...\n";
sleep(30); // Wait 30 seconds before checking again
} elseif ($status === 'failure' && $this->retryCount < $this->maxRetries) {
$this->retryCount++;
$nextState = 'copyData'; // Retry the operation
echo "Retrying... Attempt $this->retryCount\n";
sleep(30);
}
} else {
// Transition based on simple conditions (* is wildcard)
$nextState = $transitions['*'];
}
// Move to the next state
$this->state = $nextState;
}
echo "Process completed.\n";
}
private function checkCopyData() {
// Simulate checking the status of the copy process
if ($this->retryCount < $this->maxRetries) {
return 'running'; // Simulate still running
}
return 'failure'; // Simulate failure after max retries
}
}
// Initialize and run the FSM
$fsm = new FSM();
$fsm->run();
?>
The output of the provided PHP code will simulate the execution of the Finite State Machine (FSM) through its states and transitions. Here's a step-by-step breakdown of what will happen when you run the code:
Initial Setup
- The process starts in the start state.
- From the start state, it transitions to the copyData state.
Transition Breakdown
1. State: start
- The FSM starts in the start state.
- Transition: start → copyData
Output : Current state: start
2. State: copyData
- The FSM moves to the CopyData state.
- Transition: copyData → waitCopyData.
Output : Current state: copyData
3. State: waitCopyData
- The FSM is now in the waitCopyData state, where it will check the status of the copy process.
- The function checkCopyData() is called, and since it's the first attempt, the retry count is 0. The checkCopyData() method returns 'running' (because the retry count is less than the max retries).
- Transition: waitCopyData → waitCopyData (it stays in the waitCopyData state and waits 30 seconds before checking again).
Output
Current state: waitCopyData
Copy data status: running
Waiting for 30 seconds...
4. State: waitCopyData (second check)
- After 30 seconds, the checkCopyData() function is called again. The retry count is still 1 (since it was less than the max retries). The method still returns 'running'.
- Transition: waitCopyData → waitCopyData again, and the FSM waits another 30 seconds.
Output
Current state: waitCopyData
Copy data status: running
Waiting for 30 seconds...
5. State: waitCopyData (third check)
- After another 30 seconds, the checkCopyData() function is called again, and now the retry count is 2 (which is equal to the max retries). The method returns 'failure' (simulating failure after the maximum retries).
- Tnsition: waitCopyData → failure.
Output
Current state: waitCopyData
Copy data status: failure
Retrying... Attempt 2
Waiting for 30 seconds...
6. State: failure
- The FSM moves to the failure state after retrying twice.
- Transition: failure → finish.
Output: Current state: failure
7. State: End Of Process
- The process completes as it transitions to the finish state.
Output : Process completed.
Complete Output
Combining all the steps above, the full output when you run the code will look like this
Current state: start
Current state: copyData
Current state: waitCopyData
Copy data status: running
Waiting for 30 seconds...
Current state: waitCopyData
Copy data status: running
Waiting for 30 seconds...
Current state: waitCopyData
Copy data status: failure
Retrying... Attempt 2
Waiting for 30 seconds...
Current state: failure
Process completed.
Explanation Of the Code
Let's understand the code in more detail.
State Initialization
In the constructor (__construct), we initialize the starting state (start) and define the state transitions in the stateTable.
Run Method
The run() method is the core logic. It loops through the states and transitions until it reaches the finish state.
State Transitions
For each state, we check the available transitions. If a transition involves checking the status of a task (e.g., checkCopyData), the method checkCopyData() is called to simulate checking the process status.
Retries and Delays
If the task is still running, we wait for 30 seconds before checking again. If the task fails, the system will retry up to 2 times before transitioning to the failure state.
Final Thoughts
Finite State Machines (FSMs) offer a structured way to handle complicated workflows and asynchronous tasks in PHP applications, ensuring reliability and scalability. At LN Webworks, we support advanced techniques like FSMs to build seamless, error-resistant applications.
Contact us today to discover how we can take your project to the next level.Our expert team is here to help you optimise your processes and deliver efficient, innovative solutions.